Headers
π§Ύ Headers Overview
π Native Header Purposes
1.
2.
3.
4.
5.
π Authentication Headers
Header | Description |
---|---|
U | Username assigned by CSQ. |
ST | Unix timestamp (valid for 30 seconds). Used as a salt for hashing. |
SH | Salted hash calculated as:sha256hex( sha256hex(password) + sha256hex(salt) ) β all lowercase hex-encoded. |
β If any of these are incorrect or expired, the server returns 401 Unauthorized
or500 Internal Server Error
.
π Response Encryption
Accept
header to define how you want the response:application/encrypt
: Forces AES-encrypted binary responses using AES/CBC/PKCS5Padding with CSQ-provided keys.application/json
: Requests plain JSON (unencrypted).β Only these two content types are supported.
Content-Type
header and returned in binary format.π§ Smart Caching via Checksum
Header | Role |
---|---|
Cache-Hash | Sent by the client, containing the last known hash. If it matches, the body isnβt re-sent. |
New-Cache-Hash | Server returns the SHA-256 checksum of the current response. |
Recommended for endpoints with large static responses. Avoid using this with dynamic or payment operations.
π Compression Support
Header | Value | Result |
---|---|---|
Accept-Encoding | gzip | Returns a compressed payload |
identity | Returns uncompressed content |
Content-Encoding
header.π₯ Required Request Headers
Header | Description |
---|---|
U | CSQ-assigned username |
ST | Current Unix timestamp |
SH | Salted hash (see above) |
X-Real-Ip | Client's origin IP |
Accept-Encoding | Compression method (gzip or identity ) |
Accept | Response format (json or encrypt ) |
Cache-Hash | Last response hash or a placeholder |
Host | Target server |
Agent | Identifies client system or app |
π€ Response Headers
Header | Meaning |
---|---|
UID | Internal request tracking ID |
U | Echoes the request's U value |
New-Cache-Hash | SHA-256 checksum of the response body |
Content-Type | Media type (application/json or application/encrypt ) |
Content-Length | Size of the response in bytes |
Date | Server-side timestamp (GMT) |
Connection | Always set to close (stateless protocol) |
How to obtain headers
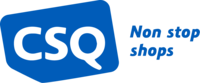
Modified atΒ 2025-07-03 18:33:44